jQuery
Overview
ZingChart offers jQuery charts by providing this wrapper for the ZingChart charting library that allows for jQuery-style use of the many different API methods and events that ZingChart has to offer. It's designed to allow maximum use of the various features with the simplest and most jQuery-esque syntax possible.
Intro
Before starting with this wrapper, please familiarize yourself with the ZingChart library. There are even several tutorials for creating your first chart with ZingChart. It should get you up to speed on how this library works.
Looking for more info? Check out any of the below tutorials to get up to speed on the ZingChart library or dig into our docs pages.
Basics
Step One is to make sure you have jQuery loaded. This wrapper won't work without it.
The ZingChart jQuery wrapper works just like normal jQuery. Each method or event is tacked on to the standard jQuery selector method. All methods should be placed inside a $(document).ready()
call to ensure the DOM is fully loaded. Here's an example of creating a ZingChart object on a div with an ID of $('#myChart')
:
$(document).ready(function() { $('#myChart').zingchart({ data: { type: 'line', series: [{ values: [1,2,5,3,9,4] }] } }); })
For the sake of brevity, the rest of the examples will omit the $(document).ready()
wrapper. That being said, you still need to include it when using this library.
All of the methods which take an object as a parameter can have it passed through directly or by reference. Both are equivalent.
Directly
$('#myChart').zingchart({ data: { type: 'bar', series: [{ values: [3,7,9,2] }] } });
Reference
var myData = { type: 'bar', series: [{ values: [3,7,9,2] }] }; $('#myChart').zingchart({data: myData});
Woohoo! Congrats! You've just made your first ZingChart. Pretty straightforward, isn't it? Now we get into the nitty gritty of the API: how to make your chart do stuff.
Chaining
One of the more user-friendly aspects of jQuery is the chaining of functions, allowing for users to specify the target once but call multiple functions that affect it. This wrapper supports chaining for any methods or events that return a jQuery object. For example, say you want to set a chart to the 3D view and resize it. Instead of calling each method on the chart separately, you could chain them like this:
$('#myChart').set3dView({'y-angle':10}).resizeChart({width:600, height:400});
$("#myChart") is now set to a 3D view and resized in just one line of code! Pat yourself on the back for saving time by using chaining. You're a rock star!
An Important Note: Many of the event functions may be very similar in name to the method functions and vice versa. This is intentional. The differentiation between the method functions and the event functions is that the method functions always start with a verb: the action they're performing, while the event functions always start with a noun: the object they're watching.
Examples
Chart Manipulation: Using Methods and Events Together
Check out this example to see how to make a chart with lots of plots into an interactive and much more legible chart:
Chart to DOM: Manipulating the DOM with Events
Learn how to manipulate the DOM through the use of ZingChart jQuery events:

DOM to Chart: Manipulating the Chart with Methods
This is a great first example if you're looking to learn how to integrate ZingChart jQuery methods with standard DOM input elements:
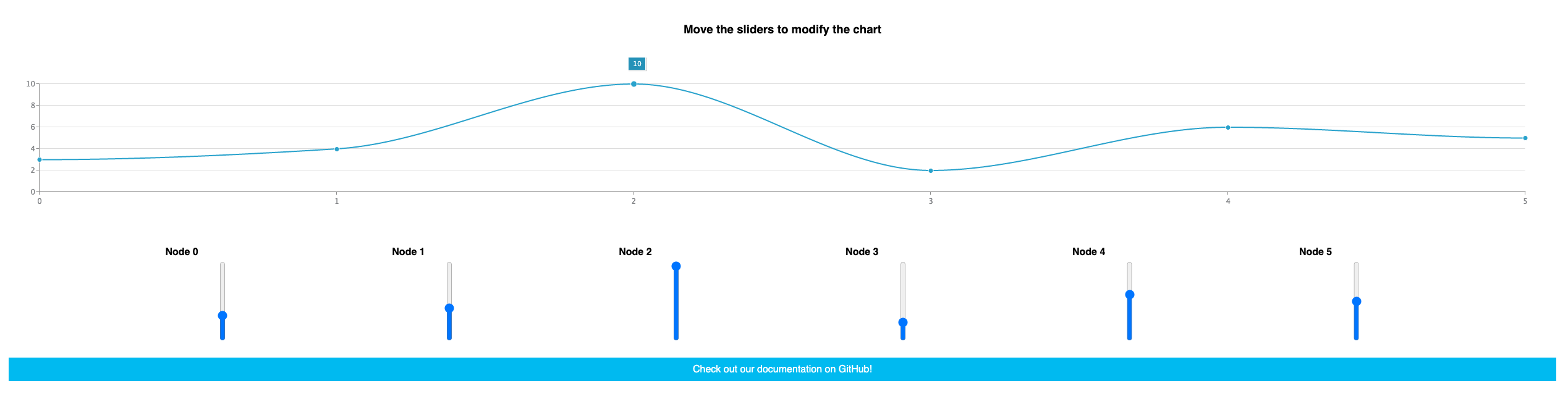
AJAX Data: Using Asynchronous Data with your Chart
No need to load your data at once. Check out this example to see how to get started with AJAX and the ZingChart jQuery wrapper:

Table to Chart: Using the Zingify Tool
Take your well-formed HTML tables and turn them into snazzy charts with ease.
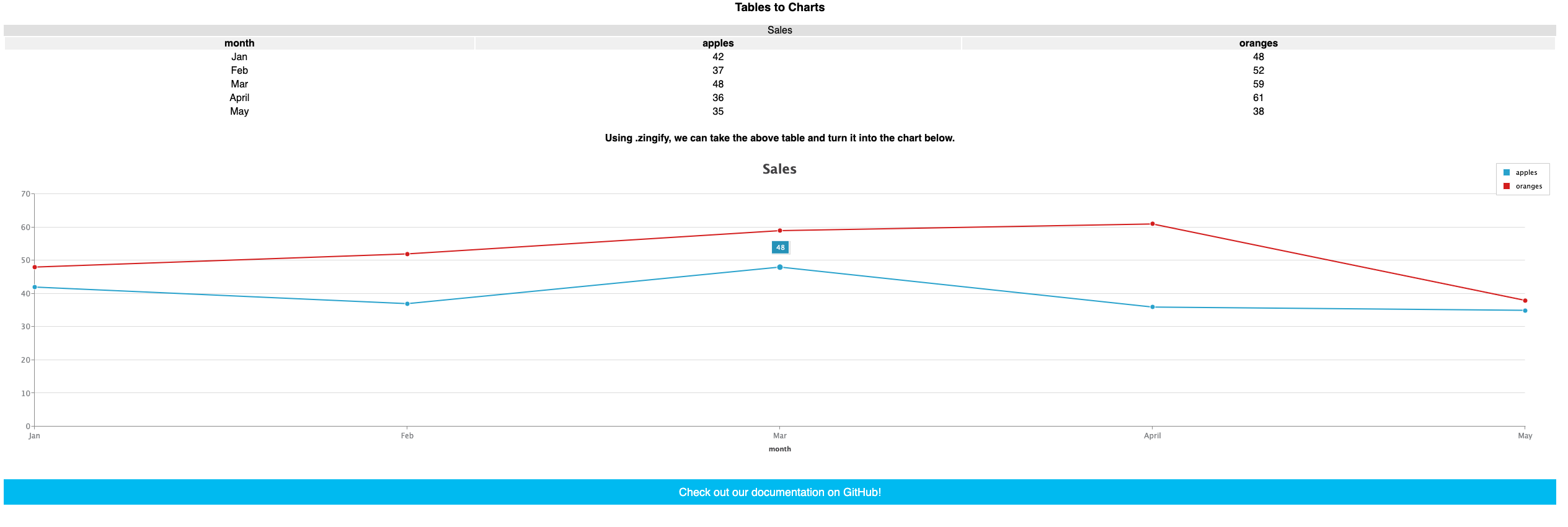